#include <stdlib.h>
#include <iostream.h>
FILE *ifp, *ofp;
int rgb_2_yuv422(FILE *ifp, FILE *ofp);
int rgb_2_yuv420(FILE *ifp, FILE *ofp);
int select_option(void);
void main()
{
int result = 0,selectoption = 0;
selectoption = select_option();
switch(selectoption)
{
case 1:
result = rgb_2_yuv422(ifp, ofp);
break;
case 2:
result = rgb_2_yuv420(ifp, ofp);
break;
default:
;
}
if (result == -1)
{
cout<< "You did not change anything!!n";
}
else if (result == 3)
{
cout<< "You convert from RGB to YUV422!!n";
}
else if (result == 4)
{
cout<< "You convert from RGB to YUV420!!n";
}
}
int rgb_2_yuv422(FILE *ifp, FILE *ofp)
{ ifp = fopen("C:\fore_cif.raw", "rb");
ofp = fopen("C:\output.raw", "wb+");
int i,j;
int R, G, B;
int Y, U, V;
unsigned char *RGBBuffer = new unsigned char[512*512*3];
unsigned char *YBuffer = new unsigned char[512*512];
unsigned char *UBuffer = new unsigned char[512*512/2];
unsigned char *VBuffer = new unsigned char[512*512/2];
unsigned char *ULine = (new unsigned char[512+2])+1;
unsigned char *VLine = (new unsigned char[512+2])+1;
ULine[-1]=ULine[512]=128;
VLine[-1]=VLine[512]=128;
fread(RGBBuffer, sizeof(char), 512*512*3, ifp);
for (i=0; i<512; ++i)
{
int RGBIndex = 3*512*i;
int YIndex = 512*i;
int UVIndex = 512*i/2;
for ( j=0; j<512; ++j)
{
R = RGBBuffer[RGBIndex++];
G = RGBBuffer[RGBIndex++];
B = RGBBuffer[RGBIndex++];
//Convert RGB to YUV
Y = (unsigned char)( ( 66 * R + 129 * G + 25 * B + 128) >> 8) + 16 ;
U = (unsigned char)( ( -38 * R - 74 * G + 112 * B + 128) >> 8) + 128 ;
V = (unsigned char)( ( 112 * R - 94 * G - 18 * B + 128) >> 8) + 128 ;
YBuffer[YIndex++] = static_cast<unsigned char>( (Y<0) ? 0 : ((Y>255) ? 255 : Y) );
VLine[j] = V;
ULine[j] = U;
}
for ( j=0; j<512; j+=2)
{
//Filter line
V = ((VLine[j-1]+2*VLine[j]+VLine[j+1]+2)>>2);
U = ((ULine[j-1]+2*ULine[j]+ULine[j+1]+2)>>2);
//Clip and copy UV to output buffer
VBuffer[UVIndex] = static_cast<unsigned char>( (V<0) ? 0 : ((V>255) ? 255 : V) );
UBuffer[UVIndex++] = static_cast<unsigned char>( (U<0) ? 0 : ((U>255) ? 255 : U) );
}
}
fwrite(YBuffer, sizeof(char), 512*512, ofp);
fwrite(VBuffer, sizeof(char), 512*512/2, ofp);
fwrite(UBuffer, sizeof(char), 512*512/2, ofp);
return 3;
fclose(ifp);
fclose(ofp);
}
int rgb_2_yuv420(FILE *ifp, FILE *ofp)
{ ifp = fopen("C:\news_cif.raw", "rb");
ofp = fopen("C:\output.raw", "wb+");
int i,j;
int R, G, B;
int Y, U, V;
unsigned char *RGBBuffer = new unsigned char[512*512*3];
unsigned char *YBuffer = new unsigned char[512*512];
unsigned char *UBuffer = new unsigned char[512*512/4];
unsigned char *VBuffer = new unsigned char[512*512/4];
int *ULine = (new int[512+2])+1;
int *VLine = (new int[512+2])+1;
ULine[-1]=ULine[512]=128;
VLine[-1]=VLine[512]=128;
int *UImage = (new int[(512+2)*(512+2)])+(512+2)+1;
int *VImage = (new int[(512+2)*(512+2)])+(512+2)+1;
fread(RGBBuffer, sizeof(char), 512*512*3, ifp);
for (i=0; i<512; ++i)
{
int RGBIndex = 3*512*i;
int YIndex = 512*i;
for ( j=0; j<512; ++j)
{
R = RGBBuffer[RGBIndex++];
G = RGBBuffer[RGBIndex++];
B = RGBBuffer[RGBIndex++];
//Convert RGB to YUV
Y = (unsigned char)( ( 66 * R + 129 * G + 25 * B + 128) >> 8) + 16 ;
U = (unsigned char)( ( -38 * R - 74 * G + 112 * B + 128) >> 8) + 128 ;
V = (unsigned char)( ( 112 * R - 94 * G - 18 * B + 128) >> 8) + 128 ;
YBuffer[YIndex++] = static_cast<unsigned char>( (Y<0) ? 0 : ((Y>255) ? 255 : Y) );
VLine[j] = V;
ULine[j] = U;
}
for ( j=0; j<512; j+=2)
{
//Filter line
VImage[i*(512+2)+j] = ((VLine[j-1]+2*VLine[j]+VLine[j+1]+2)>>2);
UImage[i*(512+2)+j] = ((ULine[j-1]+2*ULine[j]+ULine[j+1]+2)>>2);
}
}
for (i=0; i<512; i+=2)
{
int UVIndex = 512*i/4;
for ( j=0; j<512; j+=2)
{
V = ((VImage[(i-1)*(512+2)+j]+2*VImage[i*(512+2)+j]+VImage[(i+1)*(512+2)+j]+2)>>2);
U = ((UImage[(i-1)*(512+2)+j]+2*UImage[i*(512+2)+j]+UImage[(i+1)*(512+2)+j]+2)>>2);
VBuffer[UVIndex] = static_cast<unsigned char>( (V<0) ? 0 : ((V>255) ? 255 : V) );
UBuffer[UVIndex++] = static_cast<unsigned char>( (U<0) ? 0 : ((U>255) ? 255 : U) );
}
}
fwrite(YBuffer, sizeof(char), 512*512, ofp);
fwrite(VBuffer, sizeof(char), 512*512/4, ofp);
fwrite(UBuffer, sizeof(char), 512*512/4, ofp);
return 4;
fclose(ifp);
fclose(ofp);
}
int select_option(void)
{
int sel = -1;
printf("1. Convert from RGB to YUV422n");
printf("2. Convert from RGB to YUV420n");
printf("Select the option and press enter key: ");
scanf(" %d", &sel);
return sel;
}
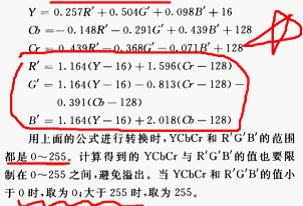
from :http://hi.baidu.com/yrworld/item/a5c548b996f9f843ba0e129c