在本节中,介绍一些一般性的JDBC背景知识。 26.3.1.1. 使用DriverManager接口连接到MySQL
在应用服务器外使用JDBC时,DriverManager类将用于管理连接的建立。
需要告诉DriverManager应与哪个JDBC驱动建立连接。完成该任务的最简单方法是:在实施了java.sql.Driver接口的类上使用Class.forName()。对于MySQL Connector/J,该类的名称是com.mysql.jdbc.Driver。采用该方法,可使用外部配置文件来提供连接到数据库时将使用的驱动类名和驱动参数。
在下面的Java代码中,介绍了在应用程序的main()方法中注册MySQL Connector/J的方式:
import java.sql.Connection;import java.sql.DriverManager;import java.sql.SQLException; // Notice, do not import com.mysql.jdbc.*// or you will have problems!(注意,不要导入com.mysql.jdbc.*,否则// 将出现问题!) public class LoadDriver { public static void main(String[] args) { try { // The newInstance() call is a work around for some // broken Java implementations Class.forName("com.mysql.jdbc.Driver").newInstance(); } catch (Exception ex) { // handle the error }}
在DriverManager中注册了驱动后,通过调用DriverManager.getConnection(),能够获得与特殊数据库相连的连接实例。
示例26.1:从DriverManager获得连接
在本示例中,介绍了从DriverManager获得连接实例的方法。对于getConnection()方法,有一些不同的特性。关于如何使用它们的更多信息,请参阅与JDK一起提供的API文档。
import java.sql.Connection;import java.sql.DriverManager;import java.sql.SQLException; ... try { Connection conn = DriverManager.getConnection("jdbc:mysql://localhost/test?user=monty&password=greatsqldb"); // Do something with the Connection .... } catch (SQLException ex) { // handle any errors System.out.println("SQLException: " + ex.getMessage()); System.out.println("SQLState: " + ex.getSQLState()); System.out.println("VendorError: " + ex.getErrorCode()); }
一旦建立了连接,它可被用于创建语句和PreparedStatements,并检索关于数据库的元数据。在下面数节内,给出了进一步的解释。
26.3.1.2. 使用语句以执行SQL
使用语句,可执行基本的SQL查询,并通过下面介绍的ResultSet类检索结果。
要想创建语句实例,应通过前面介绍的DriverManager.getConnection()或DataSource.getConnection()方法之一,在检索的连接对象上调用createStatement()方法。
一旦拥有了语句实例,可以与希望使用的SQL一起通过调用executeQuery(String)方法执行SELECT查询。
要想更新数据库中的数据,可使用executeUpdate(String SQL)方法。该方法将返回受更新语句影响的行数。
如果你事先不清楚SQL语句是SELECT或UPDATE/INSERT,应使用execute(String SQL)方法。如果SQL查询是SELECT,本方法将返回“真”,如果SQL查询是UPDATE/INSERT/DELETE,本方法将返回“假”。如果是SELECT查询,能够通过调用getResultSet()方法检索结果。如果是UPDATE/INSERT/DELETE查询,能够通过在语句实例上调用getUpdateCount()检索受影响的行计数。
示例26.2:使用java.sql.Statement执行SELECT查询
// assume conn is an already created JDBC connectionStatement stmt = null;ResultSet rs = null;try { stmt = conn.createStatement(); rs = stmt.executeQuery("SELECT foo FROM bar"); // or alternatively, if you don't know ahead of time that // the query will be a SELECT... if (stmt.execute("SELECT foo FROM bar")) { rs = stmt.getResultSet(); } // Now do something with the ResultSet ....} finally { // it is a good idea to release // resources in a finally{} block // in reverse-order of their creation // if they are no-longer needed if (rs != null) { try { rs.close(); } catch (SQLException sqlEx) { // ignore } rs = null; } if (stmt != null) { try { stmt.close(); } catch (SQLException sqlEx) { // ignore } stmt = null; }}
26.3.1.3. 使用CallableStatements以执行存储程序
从MySQL服务器5.0版开始,与Connector/J 3.1.1或更新版本一起使用时,可完全实现java.sql.CallableStatement接口,但getParameterMetaData()方法例外。
在MySQL参考手册的“存储程序和函数”一节中,介绍了MySQL存储程序的语法。
通过JDBC的CallableStatement接口,Connector/J指明了存储程序的功能。
在下面的示例中,给出了1个存储程序,它返回增量为1的inOutParam的值,并通过inputParam传递了作为ResultSet的字符串。
示例26.3. 存储程序示例
CREATE PROCEDURE demoSp(IN inputParam VARCHAR(255), INOUT inOutParam INT)BEGIN DECLARE z INT; SET z = inOutParam + 1; SET inOutParam = z; SELECT inputParam; SELECT CONCAT('zyxw', inputParam);END
要想与Connector/J一起使用demoSp,可采取下述步骤:
1. 使用Connection.prepareCall()准备可调用语句。
注意,必须使用JDBC转义语法,而且必须使用包含占位符的圆括号:
示例26.4. 使用Connection.prepareCall()
导入java.sql.CallableStatement: ... // // Prepare a call to the stored procedure 'demoSp' // with two parameters // // Notice the use of JDBC-escape syntax ({call ...}) // CallableStatement cStmt = conn.prepareCall("{call demoSp(?, ?)}"); cStmt.setString(1, "abcdefg");注释:
Connection.prepareCall()是一种开销很大的方法,原因在于驱动程序执行的支持输出参数的元数据检索。出于性能方面的原因,应在你的代码中再次使用CallableStatement实例,通过该方式,使对Connection.prepareCall()的不必要调用降至最低。
2. 注册输出参数(如果有的话)
为了检索输出参数的值(创建存储程序时指定为OUT或INOUT的参数),JDBC要求在CallableStatement接口中使用各种registerOutputParameter()方法来执行语句之前指定它们:
示例26.5. 注册输出参数
导入java.sql.Types: ... // // Connector/J supports both named and indexed // output parameters. You can register output // parameters using either method, as well // as retrieve output parameters using either // method, regardless of what method was // used to register them. // // The following examples show how to use // the various methods of registering // output parameters (you should of course // use only one registration per parameter). // // // Registers the second parameter as output // cStmt.registerOutParameter(2); // // Registers the second parameter as output, and // uses the type 'INTEGER' for values returned from // getObject() // cStmt.registerOutParameter(2, Types.INTEGER); // // Registers the named parameter 'inOutParam' // cStmt.registerOutParameter("inOutParam"); // // Registers the named parameter 'inOutParam', and // uses the type 'INTEGER' for values returned from // getObject() // cStmt.registerOutParameter("inOutParam", Types.INTEGER); ...
3. 设置输入参数(如果有的话)
输入以及输入/输出参数是作为PreparedStatement对象而设置的。但是,CallableStatement也支持按名称设置参数:
示例26.6. 设置CallableStatement输入参数
... // // Set a parameter by index // cStmt.setString(1, "abcdefg"); // // Alternatively, set a parameter using // the parameter name // cStmt.setString("inputParameter", "abcdefg"); // // Set the 'in/out' parameter using an index // cStmt.setInt(2, 1); // // Alternatively, set the 'in/out' parameter // by name // cStmt.setInt("inOutParam", 1); ...
4. 执行CallableStatement,并检索任何结果集或输出参数。
尽管CallableStatement支持调用任何语句执行方法(executeUpdate(),executeQuery()或execute()),最灵活的方法是调用execute(),这是因为,采用该方法,你无需事先知道存储程序是否将返回结果集:
示例26.7. 检索结果和输出参数值
... boolean hadResults = cStmt.execute(); // // Process all returned result sets // while (hadResults) { ResultSet rs = cStmt.getResultSet(); // process result set ... hadResults = cStmt.getMoreResults(); } // // Retrieve output parameters // // Connector/J supports both index-based and // name-based retrieval // int outputValue = cStmt.getInt(1); // index-based outputValue = cStmt.getInt("inOutParam"); // name-based ...
26.3.1.4. 检索AUTO_INCREMENT列的值
在JDBC API 3.0版之前,没有从支持“自动增量”或ID列的数据库中检索关键值的标准方法。对于针对MySQL的早期JDBC驱动,总是不得不在语句接口上使用特定的MySQL方法,或在向拥有AUTO_INCREMENT关键字的表发出“INSERT后”发出“SELECT LAST_INSERT_ID()”。特殊的MySQL方法调用是不可移植的,而且对于发出“SELECT”以获取AUTO_INCREMENT关键字的值来说,需要对数据库执行另一往返操作,其效率不高。在下面的代码片段中,介绍了检索AUTO_INCREMENT值的三种不同方式。首先,介绍了JDBC-3.0新方法“getGeneratedKeys()”的使用方式,当你需要检索AUTO_INCREMENT关键字并访问JDBC-3.0,它是目前的首选方法。在第2个示例中,介绍了使用标准“SELECT LAST_INSERT_ID()”查询检索相同值的方法。在最后一个示例中,介绍了使用“insertRow()”方法时,用可更新结果集检索AUTO_INCREMENT值的方式。 示例26.8. 使用Statement.getGeneratedKeys()检索AUTO_INCREMENT列的值
Statement stmt = null; ResultSet rs = null; try { // // Create a Statement instance that we can use for // 'normal' result sets assuming you have a // Connection 'conn' to a MySQL database already // available stmt = conn.createStatement(java.sql.ResultSet.TYPE_FORWARD_ONLY, java.sql.ResultSet.CONCUR_UPDATABLE); // // Issue the DDL queries for the table for this example // stmt.executeUpdate("DROP TABLE IF EXISTS autoIncTutorial"); stmt.executeUpdate( "CREATE TABLE autoIncTutorial (" + "priKey INT NOT NULL AUTO_INCREMENT, " + "dataField VARCHAR(64), PRIMARY KEY (priKey))"); // // Insert one row that will generate an AUTO INCREMENT // key in the 'priKey' field // stmt.executeUpdate( "INSERT INTO autoIncTutorial (dataField) " + "values ('Can I Get the Auto Increment Field?')", Statement.RETURN_GENERATED_KEYS); // // Example of using Statement.getGeneratedKeys() // to retrieve the value of an auto-increment // value // int autoIncKeyFromApi = -1; rs = stmt.getGeneratedKeys(); if (rs.next()) { autoIncKeyFromApi = rs.getInt(1); } else { // throw an exception from here } rs.close(); rs = null; System.out.println("Key returned from getGeneratedKeys():" + autoIncKeyFromApi);} finally { if (rs != null) { try { rs.close(); } catch (SQLException ex) { // ignore } } if (stmt != null) { try { stmt.close(); } catch (SQLException ex) { // ignore } }}
示例26.9. 使用SELECT LAST_INSERT_ID()检索AUTO_INCREMENT列的值
Statement stmt = null; ResultSet rs = null; try { // // Create a Statement instance that we can use for // 'normal' result sets. stmt = conn.createStatement(); // // Issue the DDL queries for the table for this example // stmt.executeUpdate("DROP TABLE IF EXISTS autoIncTutorial"); stmt.executeUpdate( "CREATE TABLE autoIncTutorial (" + "priKey INT NOT NULL AUTO_INCREMENT, " + "dataField VARCHAR(64), PRIMARY KEY (priKey))"); // // Insert one row that will generate an AUTO INCREMENT // key in the 'priKey' field // stmt.executeUpdate( "INSERT INTO autoIncTutorial (dataField) " + "values ('Can I Get the Auto Increment Field?')"); // // Use the MySQL LAST_INSERT_ID() // function to do the same thing as getGeneratedKeys() // int autoIncKeyFromFunc = -1; rs = stmt.executeQuery("SELECT LAST_INSERT_ID()"); if (rs.next()) { autoIncKeyFromFunc = rs.getInt(1); } else { // throw an exception from here } rs.close(); System.out.println("Key returned from " + "'SELECT LAST_INSERT_ID()': " + autoIncKeyFromFunc);} finally { if (rs != null) { try { rs.close(); } catch (SQLException ex) { // ignore } } if (stmt != null) { try { stmt.close(); } catch (SQLException ex) { // ignore } }}
示例26.10. 在可更新的ResultSets中检索AUTO_INCREMENT列的值
Statement stmt = null; ResultSet rs = null; try { // // Create a Statement instance that we can use for // 'normal' result sets as well as an 'updatable' // one, assuming you have a Connection 'conn' to // a MySQL database already available // stmt = conn.createStatement(java.sql.ResultSet.TYPE_FORWARD_ONLY, java.sql.ResultSet.CONCUR_UPDATABLE); // // Issue the DDL queries for the table for this example // stmt.executeUpdate("DROP TABLE IF EXISTS autoIncTutorial"); stmt.executeUpdate( "CREATE TABLE autoIncTutorial (" + "priKey INT NOT NULL AUTO_INCREMENT, " + "dataField VARCHAR(64), PRIMARY KEY (priKey))"); // // Example of retrieving an AUTO INCREMENT key // from an updatable result set // rs = stmt.executeQuery("SELECT priKey, dataField " + "FROM autoIncTutorial"); rs.moveToInsertRow(); rs.updateString("dataField", "AUTO INCREMENT here?"); rs.insertRow(); // // the driver adds rows at the end // rs.last(); // // We should now be on the row we just inserted // int autoIncKeyFromRS = rs.getInt("priKey"); rs.close(); rs = null; System.out.println("Key returned for inserted row: " + autoIncKeyFromRS);} finally { if (rs != null) { try { rs.close(); } catch (SQLException ex) { // ignore } } if (stmt != null) { try { stmt.close(); } catch (SQLException ex) { // ignore } }}
运行上面的示例代码时,将获得下述输出:从getGeneratedKeys():返回的键, 从“SELECT LAST_INSERT_ID():”返回1个键, 从插入的行返回1个键。请注意,有些时候,使用“SELECT LAST_INSERT_ID()”查询十分复杂,原因在于函数值与连接相关。因此,如果在相同连接上存在其他查询,函数值将被覆盖。另一方面,“getGeneratedKeys()”方法是由语句实例确定的,因此,即使在相同连接上存在其他查询也能使用它,但在相同语句实例上存在其他查询时则不能使用。 26.3.2. 安装 Connector/J
26.3.2.1. 所需的软件版本 26.3.2.2. 升级旧版本
请按照下述说明安装Connector/J。 26.3.2.1. 所需的软件版本
26.3.2.1.1. 支持的Java版本 26.3.2.1.2. MySQL服务器版本指南 26.3.2.1.3. 安装驱动程序并配置CLASSPATH
26.3.2.1.1. 支持的Java版本
MySQL Connector/J支持Java-2 JVMs,包括JDK-1.2.x、JDK-1.3.x、JDK-1.4.x和JDK-1.5.x,并需要JDK-1.4.x或更新的版本进行编译(而不是运行)。MySQL Connector/J不支持JDK-1.1.x或JDK-1.0.x。
由于实现了java.sql.Savepoint,Connector/J 3.1.0和更新版本不会运行在早于1.4版的JDK上,除非关闭了类验证器(-Xverify:none),这是因为,类验证器将试图加载用于java.sql.Savepoint的类定义,除非使用了savepoint功能,否则驱动程序不会访问类验证器。
早于1.4.x版的JVM上,不能使用Connector/J 3.1.0或更高版本提供的新缓冲功能,这是因为该功能依赖在JDK-1.4.0中首次提供的java.util.LinkedHashMap。
26.3.2.1.2. MySQL服务器版本指南
MySQL Connector/J支持所有著名的MySQL服务器版本。某些特性(外键,可更新结果集)需要更新的MySQL版本才能工作。
与MySQL服务器4.1版或更高版本建立连接时,最好使用MySQL Connector/J 3.1版,这是因为它全面支持较新版本的服务器提供的特性,包括Unicode字符、视图、存储程序和服务器端预处理语句。
尽管3.0版Connector/J能够与MySQL服务器4.1或更高版本建立连接,但由于实现了Unicode字符和新的鉴定机制,将无法更新Connector/J 3.0以支持当前和未来服务器版本中提供的新特性。
26.3.2.1.3. 安装驱动程序并配置CLASSPATH
MySQL Connector/J是以.zip或.tar.gz形式分发的,其中包含源码、类文件、以及仅为“二进制”.jar的类文件(名为mysql-connector-java-[version]-bin.jar),从Connector/J 3.1.8开始,驱动程序的“调试版”位于名为“mysql-connector-java-[version]-bin-g.jar”的文件中。
从Connector/J 3.1.9开始,我们不再单独提供.class文件,仅在与驱动程序一起提供的JAR文件中提供它们。
不应使用驱动程序的“调试版”,除非是在向MySQL AB通报问题或缺陷时需要用到它,这是因为“调试版”不是为生产环境下的运行而设计的,如果使用它,会对性能造成负面影响。二进制代码的调试取决于Aspect/J运行时库,该库位于与Connector/J分发版一起提供的src/lib/aspectjrt.jar文件中。
需要使用恰当的GUI或命令行使用工具来解开分发文件(例如,用于.zip文件的WinZip,以及用于.tar.gz文件的“tar”)。由于在分发版中可能存在长文件名,我们采用了GNU tar档案格式。需要使用GNU tar(或能理解GNU tar档案格式的其他应用程序)来解开分发版的.tar.gz文件。
一旦解包了分发版档案文件,可以将mysql-connector-java-[version]-bin.jar放在你的类路径中,或是在你的CLASSPATH环境变量中添加它的完整路径,或是在启动JVM(Java虚拟机)时用命令行开关“-cp”直接指定它,通过该方式安装驱动。
如果你打算用JDBC DriverManager来使用驱动,可使用“com.mysql.jdbc.Driver”,将其用作实施了“java.sql.Driver”类。
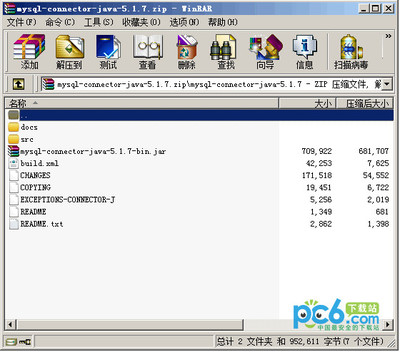
示例26.11. 设置Unix环境下的CLASSPATH
在Unix环境下,下述命令用于“csh”:
$ setenv CLASSPATH /path/to/mysql-connector-java-[version]-bin.jar:$CLASSPATH
可以将上述命令添加到恰当的、用于登录shell的启动文件中,从而使得所有的Java应用程序均能使用MySQL Connector/J。
如果希望与诸如Tomcat或Jboss等应用服务器一起使用MySQL Connector/J,应仔细阅读供应商提供的文档,以了解如何配置第三方类库的更多信息,这是因为大多数应用服务器均会忽略CLASSPATH环境变量。在“与J2EE和其他Java框架一起使用 Connector/J”一节中,给出了针对一些J2EE应用服务器的配置示例,但是,对于特定的应用服务器,JDBC连接池配置信息的权威信息源是该应用服务器的文档。
如果你准备开发小服务程序和/或JSP,而且你的应用服务器是J2EE兼容的,可以将驱动的.jar文件放到webapp的WEB-INF/lib子目录下,在J2EE Web应用程序中,这是第三方类库的标准位置。
如果你的J2EE应用服务器支持或要求,也可以使用com.mysql.jdbc.jdbc2.optional可选软件包中的MysqlDataSource或MysqlConnectionPoolDataSource类。多种MysqlDataSource类均支持下述参数(通过标准的“Set”存取器):
· user
· password
· serverName(参见前面关于故障切换主机的章节)
· databaseName
· port